❗
This SDK is currently in beta. Please report any issues you encounter by creating an issue in the aptos-labs/aptos-dotnet-sdk repository.
Getting Started
If you have not already installed the Aptos .NET SDK, follow one of the guides below to get started.
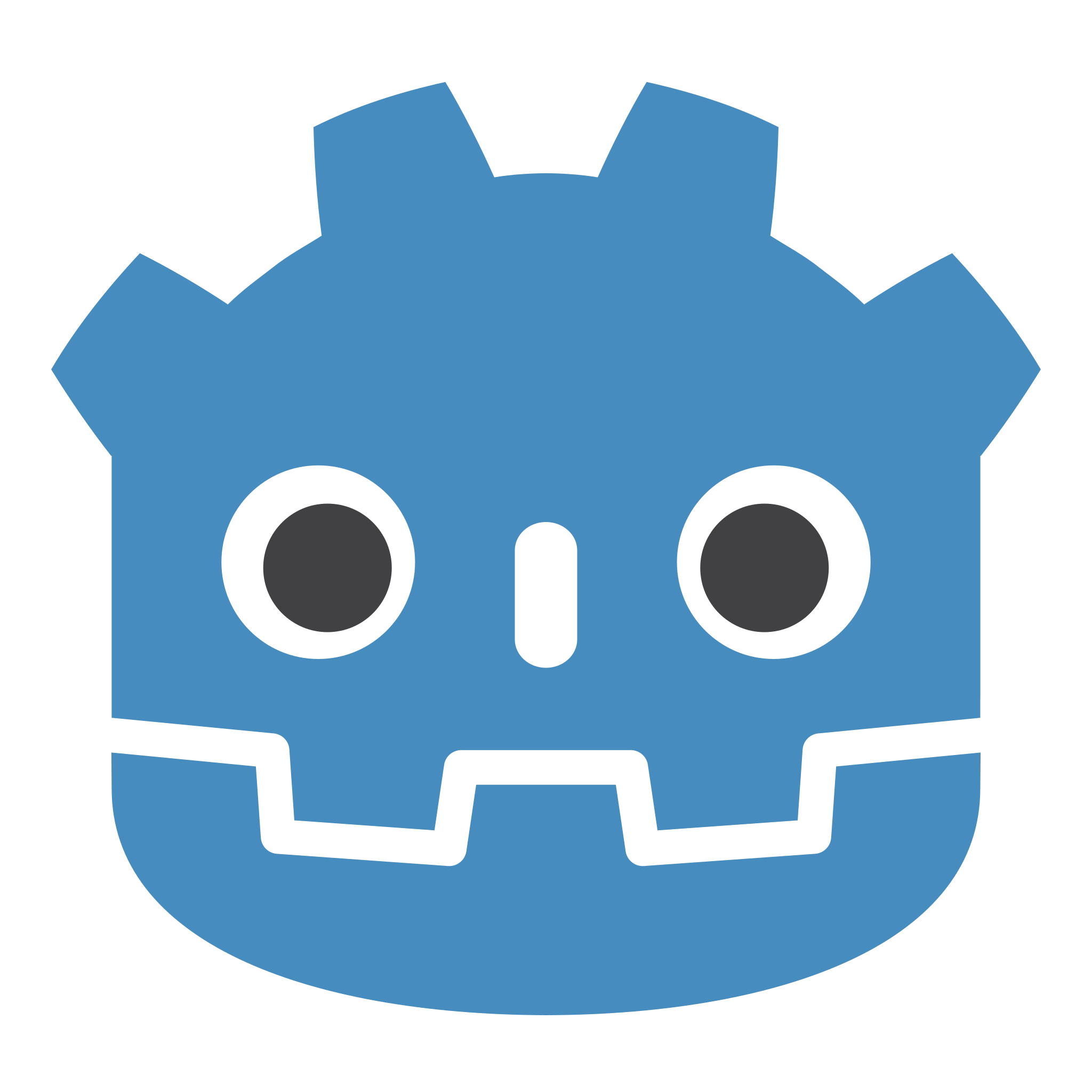
Godot Integration →
Integrate the Aptos .NET SDK with a Godot project.
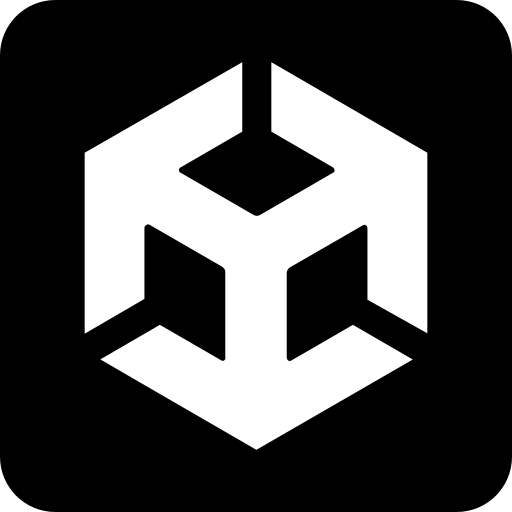
Unity SDK →
Integrate the Aptos .NET SDK with a Unity project.
Set up your AptosClient
Set up your Aptos client by adding the Aptos
namespace and instantiating an AptosClient
. You can use a predefined
configuration from Networks
or configuring your own.
Program.cs
using Aptos;
class Program
{
static void Main(string[] args)
{
var config = new AptosConfig(Aptos.Networks.Mainnet);
var client = new AptosClient(config);
}
}
Query the Blockchain
Now that you have the client setup, you can query the blockchain!
Program.cs
using Aptos;
class Program
{
static void Main(string[] args)
{
var config = new AptosConfig(Aptos.Networks.Mainnet);
var client = new AptosClient(config);
var ledgerInfo = client.Block.GetLedgerInfo();
Console.WriteLine(ledgerInfo.BlockHeight);
}
}
Sign and Submit Transactions
To interact with the blockchain, you will need to create a signer and build a transaction.
Program.cs
using Aptos;
class Program
{
static void Main(string[] args)
{
var config = new AptosConfig(Aptos.Networks.Mainnet);
var client = new AptosClient(config);
// 1. Create a signer
var signer = Account.Generate();
// 2. Build the transaction
var transaction = await client.Transaction.Build(
sender: account,
data: new GenerateEntryFunctionPayloadData(
function: "0x1::aptos_account::transfer_coins",
typeArguments: ["0x1::aptos_coin::AptosCoin"],
functionArguments: [account.Address, "100000"]
)
);
// 3. Sign and submit the transaction
var pendingTransaction = client.Transaction.SignAndSubmitTransaction(account, transaction);
// 4. (Optional) Wait for the transaction to be committed
var committedTransaction = await client.Transaction.WaitForTransaction(pendingTransaction);
}
}
Smart Contract View Functions
Call view functions to query smart contracts.
Program.cs
using Aptos;
class Program
{
static void Main(string[] args)
{
var config = new AptosConfig(Aptos.Networks.Mainnet);
var client = new AptosClient(config);
// Call the view function by specifying the function name, arguments, and type arguments
var values = await client.Contract.View(
new GenerateViewFunctionPayloadData(
function: "0x1::coin::name",
functionArguments: [],
typeArguments: ["0x1::aptos_coin::AptosCoin"]
)
);
// Returns a list of return values: ["Aptos Coin"]
Console.WriteLine("APT Name: " + values[0]);
}
}